Toon Titles: Space Bear
The more I dive into using the GSAP library for my Toon Title animations, the more I enjoy it. Here’s another Toon Title, this time based on Space Bear, a Yogi Bear cartoon from February 1960. I learned some new things while making this Toon Title, including how to use GSAP’s timeline feature.
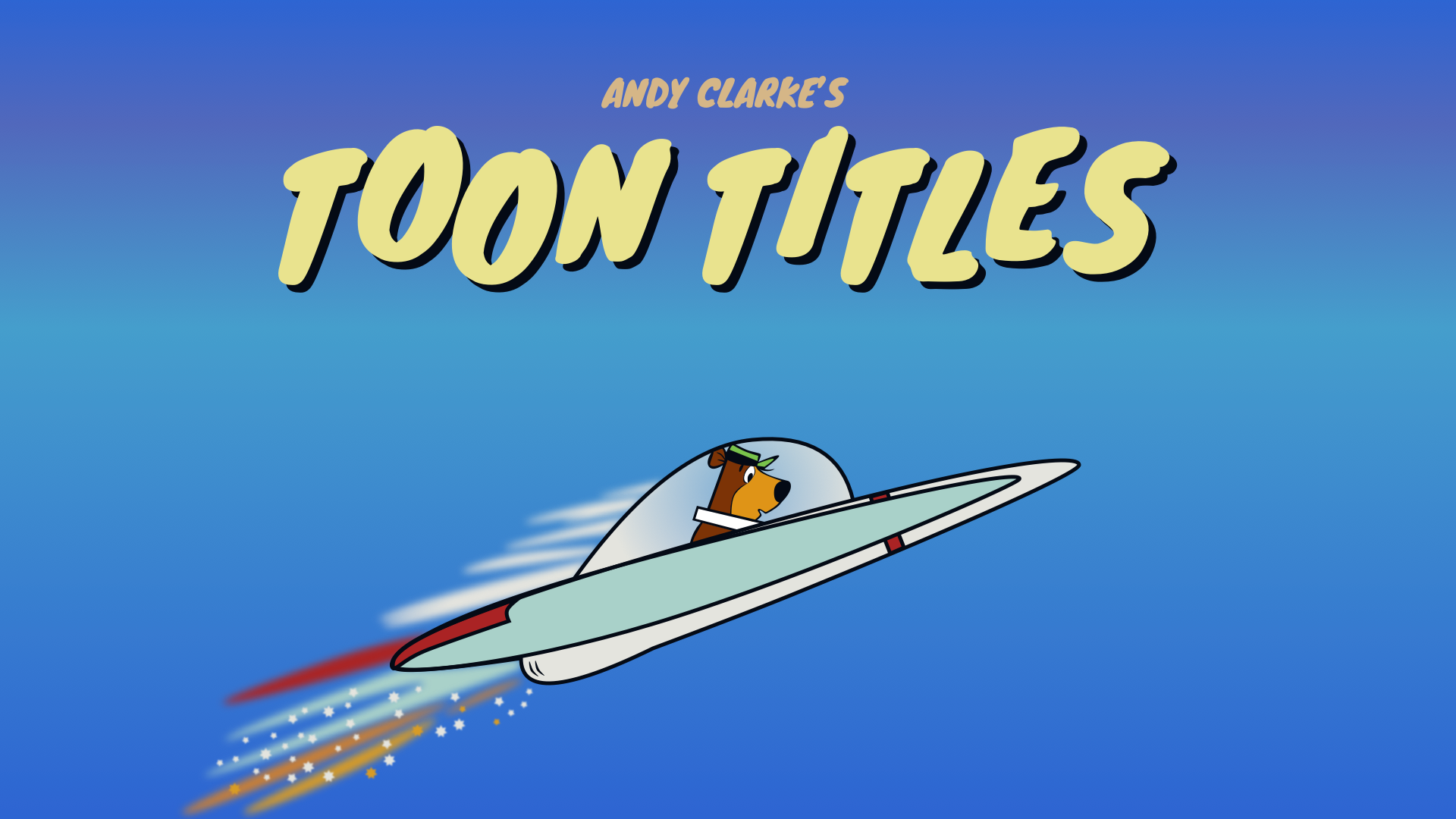
This time, Yogi’s in an SVG spaceship group which includes him, several paths which create the impression of movement, and some twinkling stars:
<g id="ship">
<g id="ship-whoosh-1" opacity=".5">…</g>
<g id="ship-whoosh-2" opacity=".5">…</g>
<g id="whoosh-stars">…</g>
<g id="yogi">…</g>
</g>
For this animation, I want:
- Yogi to fly across a motion path
- Then fly across another motion path
- Stars to twinkle in a random-looking pattern
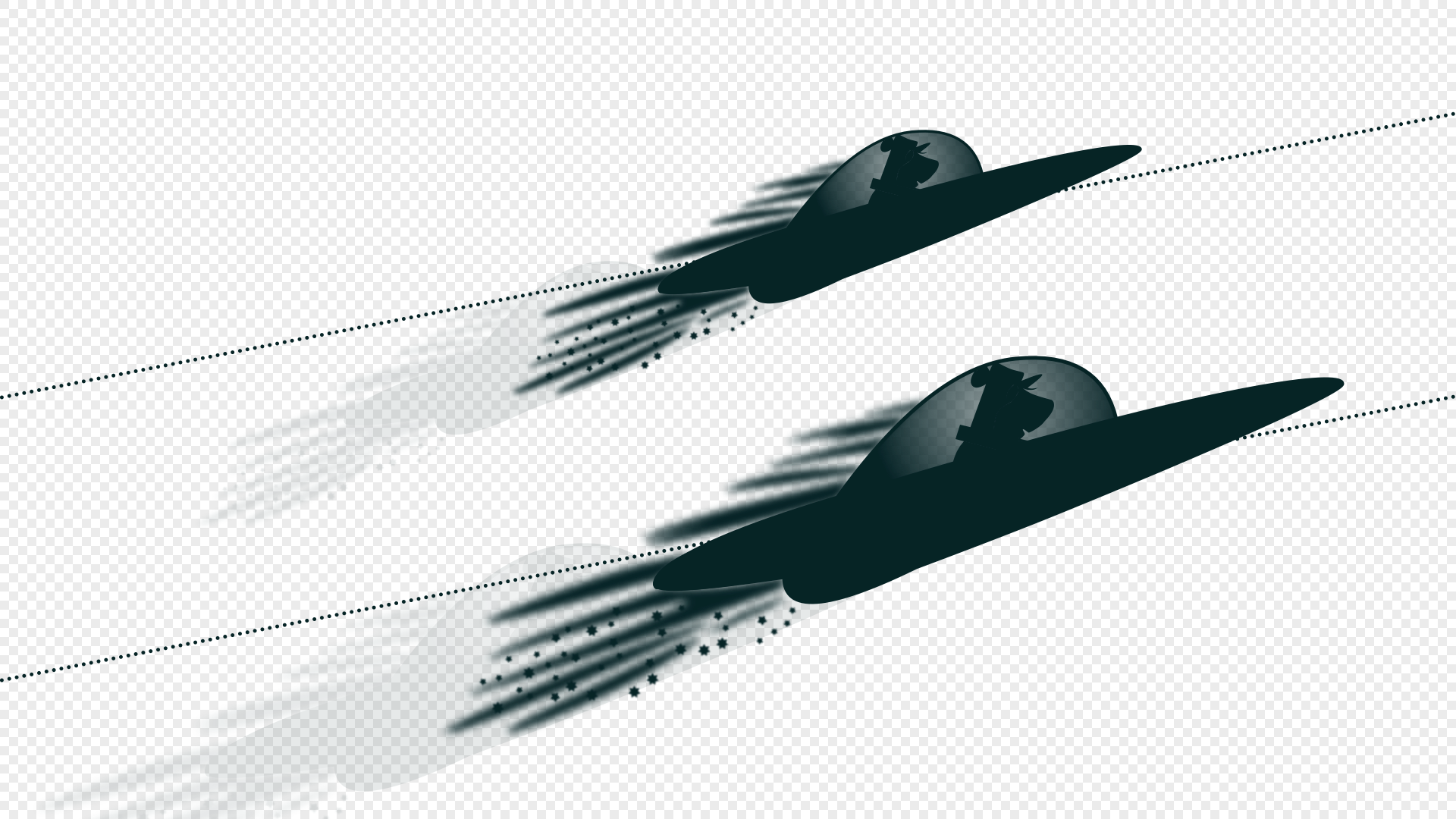
So I need two motion paths in my SVG, and I set their opacity to 1
during production, but make them invisible while I’m finished:
<path id="motion-path-1" d="…" opacity="0"/>
<path id="motion-path-2" d="…" opacity="0"/>
First, I linked to the GSAP script plus the additional motion path plug-in:
<script src="/gsap.min.js"></script>
<script src="/MotionPathPlugin.min.js"></script>
GSAP timelines are a straightforward way to control multiple animations. They make it easier to build animation sequences without manually adding delays between each one. For example, without a timeline, Yogi’s ship animation might look like:
gsap.to("#ship", { …, duration: 8 });
gsap.to("#ship", { …, delay: 1 });
This isn’t too much of a problem with just two animations, but adjusting delays would quickly become tedious if there were many more. So instead, I created a timeline called “ship” and applied that to my ship group. There are two animations in this timeline:
<script>
shipTl.to("#ship", { … }
} )
.to("#ship", { … }
} );
</script>
Then, I attached a motion path to each, plus an eight-second duration:
shipTl.to("#ship", {
duration: 8,
motionPath: {
path: "#motion-path-1" }
}
} )
.to("#ship", {
duration: 8,
motionPath: {
path: "#motion-path-2" }
}
} );
During the first animation, the ship is normal size. I want it to appear further away when it comes around again, so I scaled it down to make it look smaller:
shipTl.to("#ship", {
…,
scale: 1 }
} )
.to("#ship", {
…,
scale: .5 }
} );
Now, Yogi’s ship makes two passes along separate motion paths, and GSAP’s timeline feature means no more setting delays between each animation.
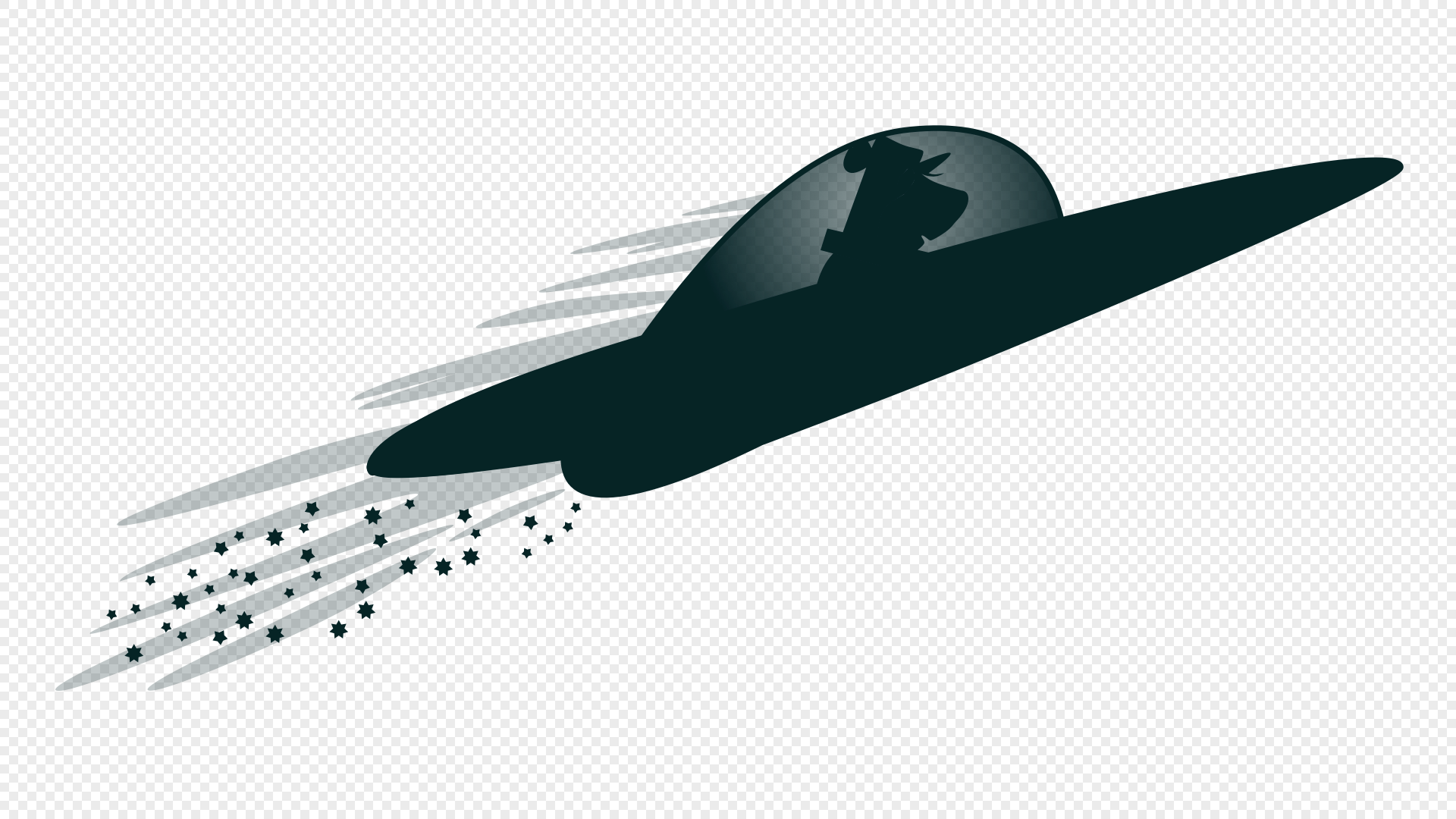
One of the most charming details in the original title card was the group of stars behind Yogi’s ship. I want to animate these stars to appear and disappear in a random-looking pattern. I created an SVG group and filled it with star-shaped paths:
<g id="whoosh-stars">
<path fill="#D79B26" d="…"/>
<path fill="#D79B26" d="…"/>
…
</g>
First, I created a constant which finds every path inside the whoosh-stars group and puts them into an array called stars
.
const stars = gsap.utils.toArray("#whoosh-stars path");
Now, I can run animations on each star one by one using a forEach()
loop. The GSAP set property sets the stars’ starting opacity to 0
, so they fade in as they twinkle:
gsap.set(stars, { opacity: 0 });
forEach()
cycles through each star in the array, one by one. Math.random()
gives a random number between 0
and 1
. duration = 0.2 + Math.random() * 0.3
means the fade in/out will take between .2s and 0.5s.
stars.forEach((star, i) => {
const delay = Math.random() * 3;
const duration = 0.2 + Math.random() * 0.3;
Like real stars, each star has random timing, so they twinkle at different times and speeds. To create the stars’ twinkling effect, I used a GSAP timeline again for each star, making it fade in and out, repeating indefinitely and with a short delay between each instance.
gsap.timeline()
builds a sequence of animations for the current star. repeat: -1
makes the timeline loop forever. yoyo: true
reverses the animation so the stars fade in and out. repeatDelay: Math.random() * 2
makes a star wait up to two seconds before repeating the animations. This makes the twinkling feel natural. The delay is the random starting delay I set earlier, which means the stars start at different times:
gsap.timeline({
repeat: -1,
yoyo: true,
repeatDelay: Math.random() * 2, delay })
Now, the stars twinkle independently, at different times, with a pause before repeating. The first animation in this timeline fades the stars to full opacity over the random duration I set earlier. To make the fade start and end smoothly, I set an easing function like a sine wave:
.to(star, {
opacity: 1,
duration,
ease: "sine.inOut" })
Then, the stars fade out again:
.to(star, {
opacity: 0,
duration,
ease: "sine.inOut" });
});
With these .to
blocks inside a timeline which repeats indefinitely and yo-yos, the stars twinkle endlessly in a beautifully random pattern.
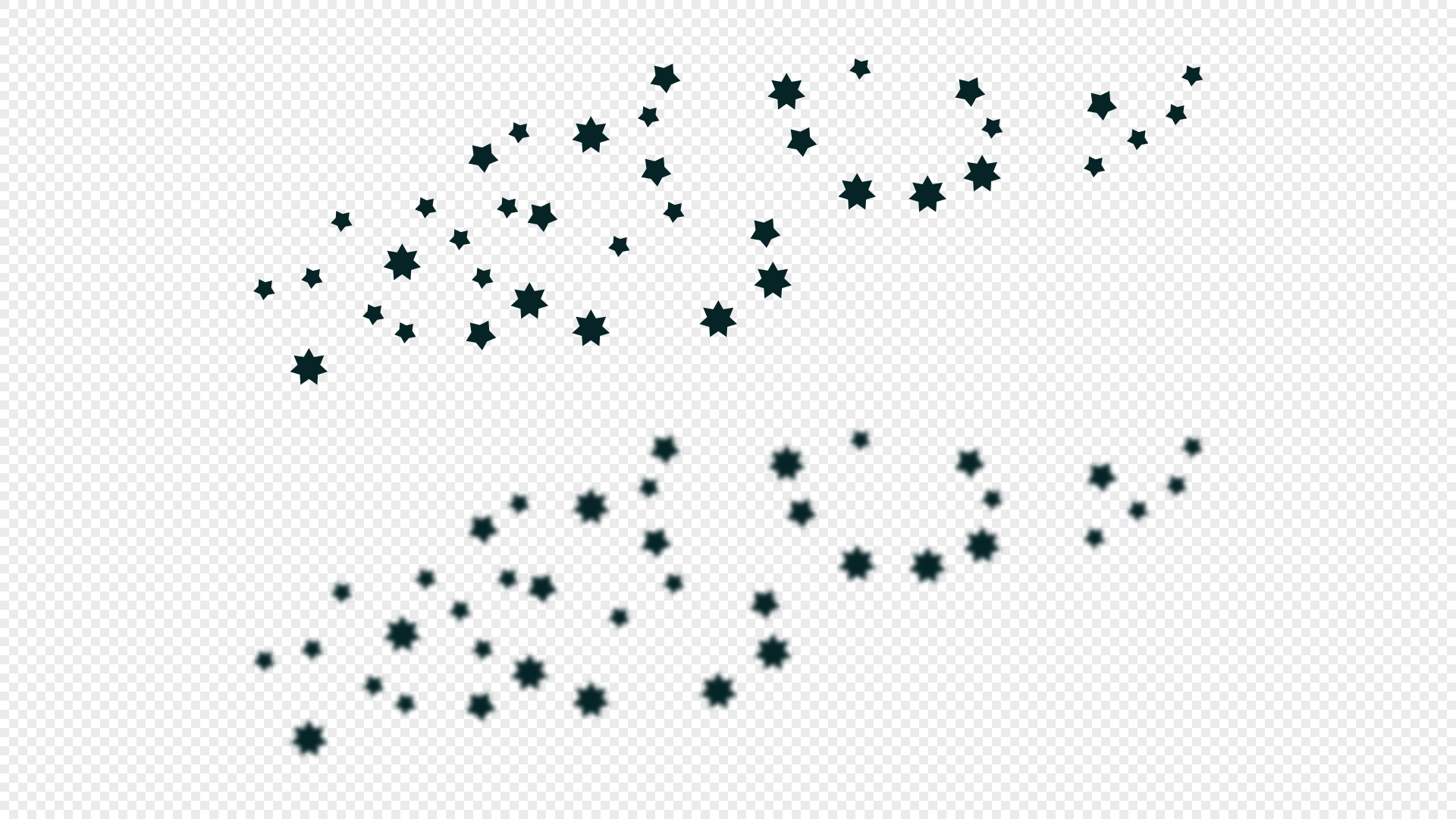
Finally, to soften the stars and help them blend into the whooshing effect, I applied a Gaussian blur filter to each one:
<g id="whoosh-stars">
<path filter="url(#whoosh-stars-blur)" d="…"/>
…
</g>
<filter id="whoosh-stars-blur" x="-50%" y="-50%" width="200%" height="200%">
<feGaussianBlur in="SourceGraphic" stdDeviation="1.5"/>
</filter>
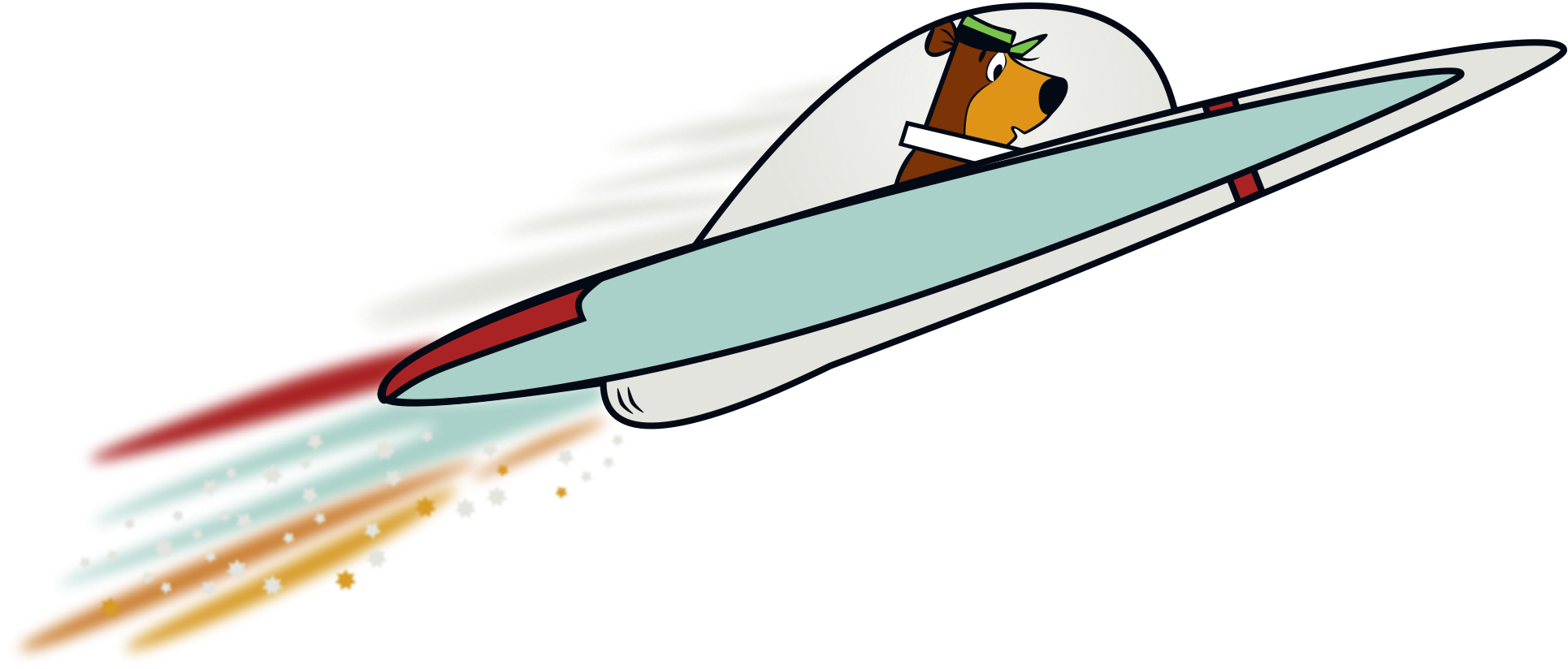
GSAP continues to surprise me with how powerful—and fun—it is to use. This Toon Title taught me how timelines can simplify complex sequences, and how even tiny details bring a scene to life. I’m still learning, but with each Toon Title I make, I feel like I’m getting closer to the kind of characterful animations I’ve always loved in classic cartoons.